CSCI 162 - Lecture 13 - Chapter 9: Trees (continued)
Instructor's Class Notes
- Binary Search Trees - In a binary search tree, the elements of the nodes can be compared with a total order semantics. These two rules are followed for every node n:
- Every element in n's left subtree is less than or equal to the element in node n.
- Every element in n's right subtree is greater than the element in node n.
Example:
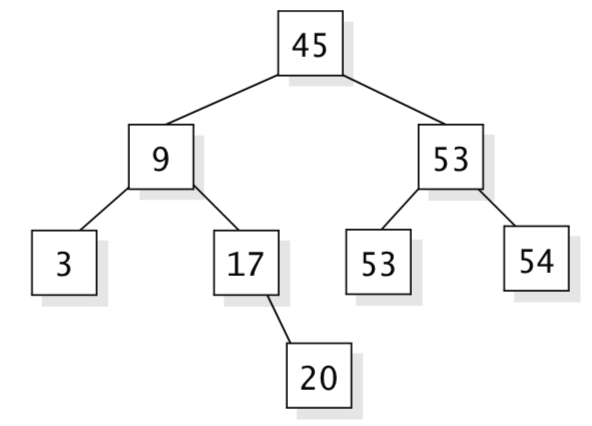
Question 1: Where might we find the number 16?
Question 2: How many comparisons to find the number 54?
Question 3: What if these same numbers were stored in an unsorted bag? In a sorted bag?
- BStree lab - For the BStree lab you must finish several methods of the BStree.java module. The BStree lab is here
Files to include:
- BStree class - You need to finish four (4) methods in this class. BStree code is here
- TreeLines.java test program - This program asks the user for a line of input and then
inserts the characters from that line into a tree and displays the resulting tree. The program
then asks for another line and then removes those characters from the tree, again, printing the results. Use this program, as-is, for testing your BStree class.
- Btree class - This like BTnode below is part of the btree package that you must
include in your project. Btree provides a class with helper methods for printing trees inorder, etc. Btree documentation and
code.
- BTnode class - This is the generic binary tree node class used by your BStree class
as well as the Btree helper class. See BTnode documentation
and code.